class: center, middle, inverse, title-slide .title[ # Shiny Part 2: Handing data in Shiny & making maps with leaflet ] .subtitle[ ##
FISH 549 ] .author[ ### Megsie Siple ] .institute[ ### NOAA AFSC - RACE/GAP ] .date[ ### 2025-03-05 (updated: 2025-03-05) ] --- # On Monday we talked about - Shiny: when should we use it? - How to build a basic Shiny app --- # Today (Wednesday) we will cover - More basic shiny principles: reactive elements, using modules, etc. - Building interactive maps with `{leaflet}` (shiny code example: *website/lectures/week_09/mapExample/*) -- - ...and we will look at some cool apps. --- class: center, middle, inverse # More Shiny basics --- # Inputs and outputs Most used input widgets: `checkboxInput()` (and `checkboxGroupInput()` allows multiple checkboxes) `radioButtons()` `actionButton()` `sliderInput()` `fileInput()` for uploading files - very helpful! `dateInput()` and `dateRangeInput()` for selecting dates --- # Inputs and outputs Most used output widgets: `plotOutput()` can be any kind of plot, includes ggplot objects `tableOutput()` `textOutput()` `uiOutput()` allows you to dynamically generate user interface components --- # Use `"showcase"` mode to show code and output side by side This option is really useful for teaching people about functionality. ``` r runApp("MyApp", display.mode = "normal") # the default runApp("MyApp", display.mode = "showcase") # shows server code alongside the ui ``` <!--  --> An example I've referred to for teaching: [K-means clustering](https://shiny.posit.co/r/gallery/start-simple/kmeans-example/), from Posit's Shiny gallery. --- # Clean up Shiny code with modules - Modules are like the Shiny equivalent of functions - You can use modules on the server side or the UI --- # Clean up Shiny code with modules .pull-left[ Here is the existing shiny code for a "basic" shiny app that draws a histogram. ``` r ui <- fluidPage( selectInput("var", "Variable", names(mtcars)), numericInput("bins", "bins", 10, min = 1), plotOutput("hist") ) server <- function(input, output, session) { data <- reactive(mtcars[[input$var]]) output$hist <- renderPlot({ hist(data(), breaks = input$bins, main = input$var) }, res = 96) } ``` ] .pull-right[ And you can turn the whole UI into a function output with something like this. `NS()` means namespace. ``` r histogramUI <- function(id) { tagList( selectInput(NS(id, "var"), "Variable", choices = names(mtcars)), numericInput(NS(id, "bins"), "bins", value = 10, min = 1), plotOutput(NS(id, "hist")) ) } ``` ] --- # Write informative error messages with `validate()` and the `shinyFeedback` pkg ``` r validate( need(input$data != "", "Please select a data set") ) ``` More here: https://shiny.posit.co/r/articles/improve/validation/ --- # Maps in Shiny with `leaflet` Leaflet is an open-source JavaScript library that makes interactive maps. It's *cool*! And the [`leaflet`](https://rstudio.github.io/leaflet/articles/leaflet.html) R package integrates well with Markdown files and Shiny apps so you can show spatial data in an interactive way. It gets fancy very fast. <img src="img/new_leaflet_example.jpeg" width="4228" style="display: block; margin: auto;" /> --- # Normal `leaflet` plots .pull-left[`leaflet` code builds plots in layers, similar to visualization tools like `ggplot2`. You build an interactive map by element: ``` r library(leaflet) leaflet() |> addTiles() |> setView( -122.31637, 47.65316, zoom = 17) # set the starting view ``` ] .pull-right[
] --- # And you can customize with different tiles and styles .pull-left[ Map code: ``` r library(leaflet) leaflet() |> addTiles(urlTemplate = "https://{s}.tile.openstreetmap.fr/hot/{z}/{x}/{y}.png") |> setView( -122.31637, 47.65316, zoom = 17) # set the starting view ``` ] .pull-right[
] --- # Demo: mackerel eggs Basic leaflet code (no shiny yet) has this 'layered' style: ``` r library(leaflet) library(gamair) # mack dataset (mackerel eggs) data(mack) x <- mack fishpal <- RColorBrewer::brewer.pal(6, name = "YlOrRd") dat2plot <- mack |> dplyr::filter(egg.dens > 0 & !is.na(vessel)) * leaflet(data = dat2plot) |> * addTiles() |> * addCircleMarkers( * ~lon, ~lat, * radius = ~ egg.dens / 30, * color = ~ fishpal, * stroke = FALSE, * fillOpacity = 0.5 * ) |> * addProviderTiles("Esri.WorldImagery") ``` --- # Demo: mackerel eggs
--- # If you already have a `{leaflet}` script that generates HTML: You can show this code inside a Shiny app with the function `renderLeaflet()` All you have to do is create a leaflet map in your server logic, like so: ``` r * var2plot <- reactive(mack[, input$which_var]) # a reactive expression * output$mymap <- renderLeaflet({ # leaflet object in output data(mack) fishpal <- colorNumeric(RColorBrewer::brewer.pal(6, name = "YlOrRd")) # This is how you call a reactive object in Shiny! * x <- var2plot() dat2plot <- mack |> dplyr::filter(egg.dens > 0 & !is.na(x)) leaflet(data = dat2plot) |> addTiles() |> addCircleMarkers( ~lon, ~lat, radius = ~ egg.dens / 30, color = ~ fishpal, stroke = FALSE, fillOpacity = 0.5 ) |> addProviderTiles("Esri.WorldImagery") }) ``` --- # Corresponding ui.R code ``` r ui <- fluidPage( theme = shinytheme("darkly"), titlePanel("Spatial data in leaflet: Mackerel eggs"), sidebarLayout( sidebarPanel( radioButtons( inputId = "which_var", label = "Which environmental variable to show:", choiceValues = c("salinity", "flow", "temp.surf"), choiceNames = c("Salinity", "Flow", "Surface temperature") ) ), mainPanel( tabsetPanel( tabPanel( "Map", leafletOutput("mymap"), p() ), tabPanel( "Correlation", plotOutput("user_corr") ), tabPanel( "Another map", leafletOutput("new_map") ))))) ``` --- class: center, middle, inverse ## website/lectures/week_09/mapExample --- class: center, middle, inverse # Some cool Shiny apps 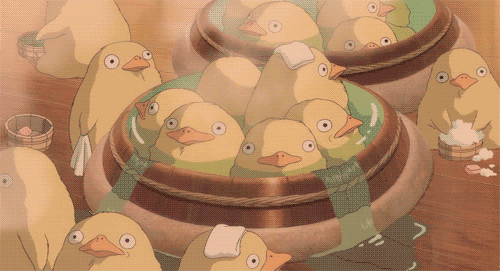 --- class: center, middle 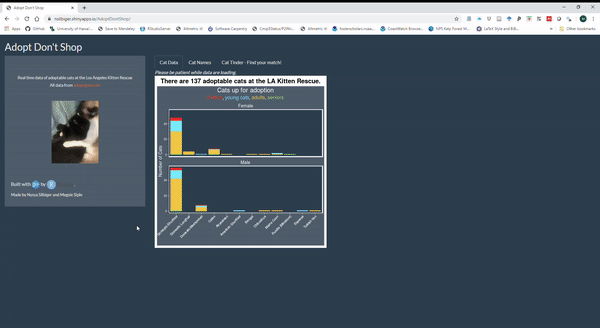 Link to the original [AdoptDontShop app](https://forum.posit.co/t/adopt-dont-shop-2020-shiny-contest-submission/59166) (it doesn't work anymore because the shelter changed its interface!) --- class: center, middle 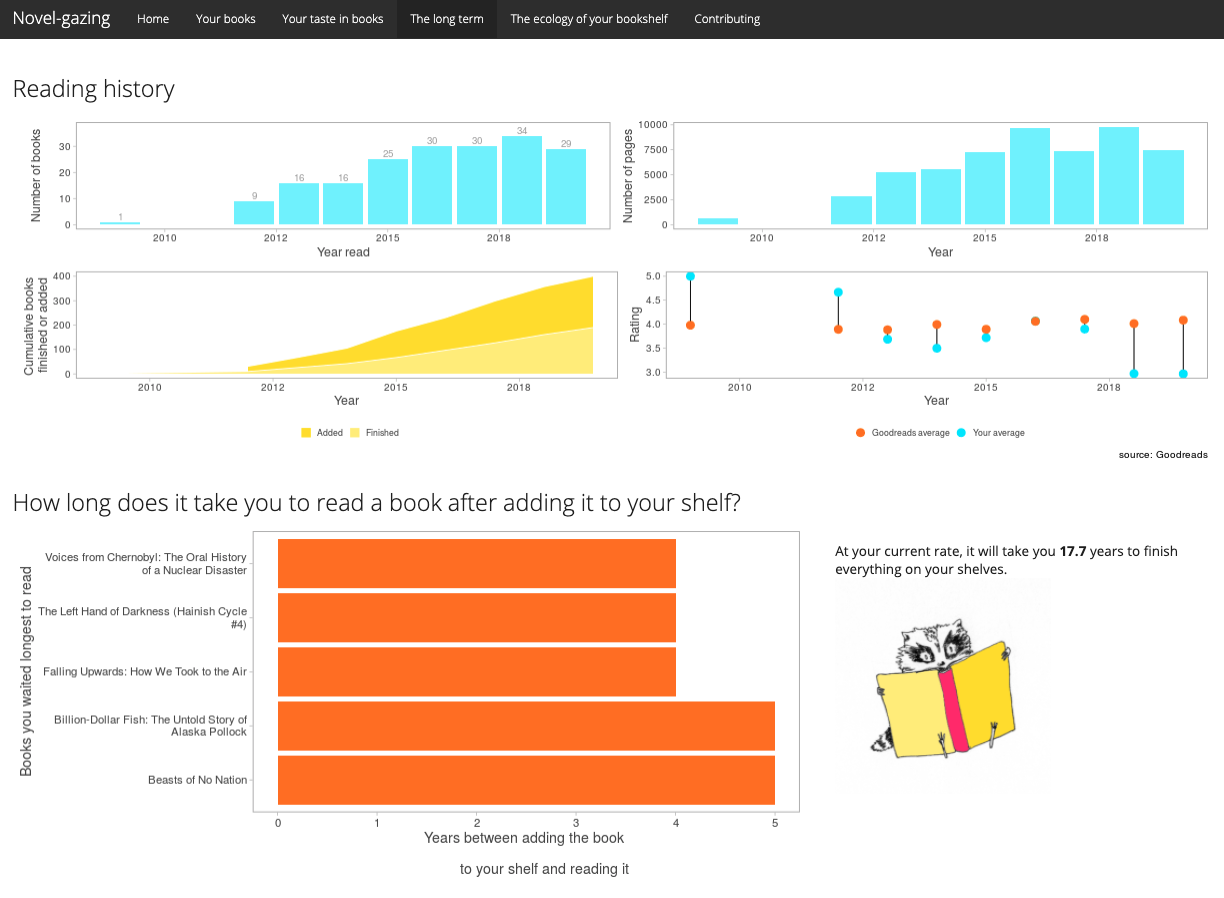 Link to the [Novel-gazing](https://msiple.shinyapps.io/NovelGazingApp/) app. --- class: center, middle # Other Shiny apps of note A cool example of how to use Shiny instead of a lengthy appendix to illustrate a point from a manuscript: [Beyond Temperature](https://heatherwelch.shinyapps.io/beyond_temperature/) by Jennifer McHenry, Heather Welch, Sarah E. Lester, and Vincent Saba An application of Shiny to aggregate data from an API (and get inspiration about data viz): [tidytuesday.rocks](https://nsgrantham.shinyapps.io/tidytuesdayrocks/) by Neal Grantham Use Shiny to provide effective data viz on large datasets: [Sentify](https://rcharlie.shinyapps.io/sentify/) by Charlie Thompson A whole gallery of great apps, complete with source code (and a great way to get recognized for your Shiny work!) All the submissions are [here](https://community.rstudio.com/c/shiny/shiny-contest/30) and the winners from the last contest (2021) are [here](https://posit.co/blog/winners-of-the-3rd-annual-shiny-contest/). --- # Fin #### Today's Shiny-relevant code tips 🍥 `Ctl-P` shortcut jumps to the close parentheses of the statement you're in. Extremely useful for Shiny dev. ☔ You can also enable rainbow parentheses in Global Options by going to `Code --> Display--> Rainbow parentheses` #### contact 📧: margaret.siple@noaa.gov If you're developing a Shiny app for a project, I would love to help you! Send me questions, issues, use code from the `shinyoverview` repo, whatever you want! *** Slides created using the R package [**xaringan**](https://github.com/yihui/xaringan). --- class: center, middle # Extra slides (deployment & getting credit for your Shiny dev work) --- # Deploying your Shiny app ## Deploy to the cloud - Sign up for a free shinyapps.io account - Install `{rsconnect}` - Configure `rsconnect` to use your account using a token from your shinyapps.io account: 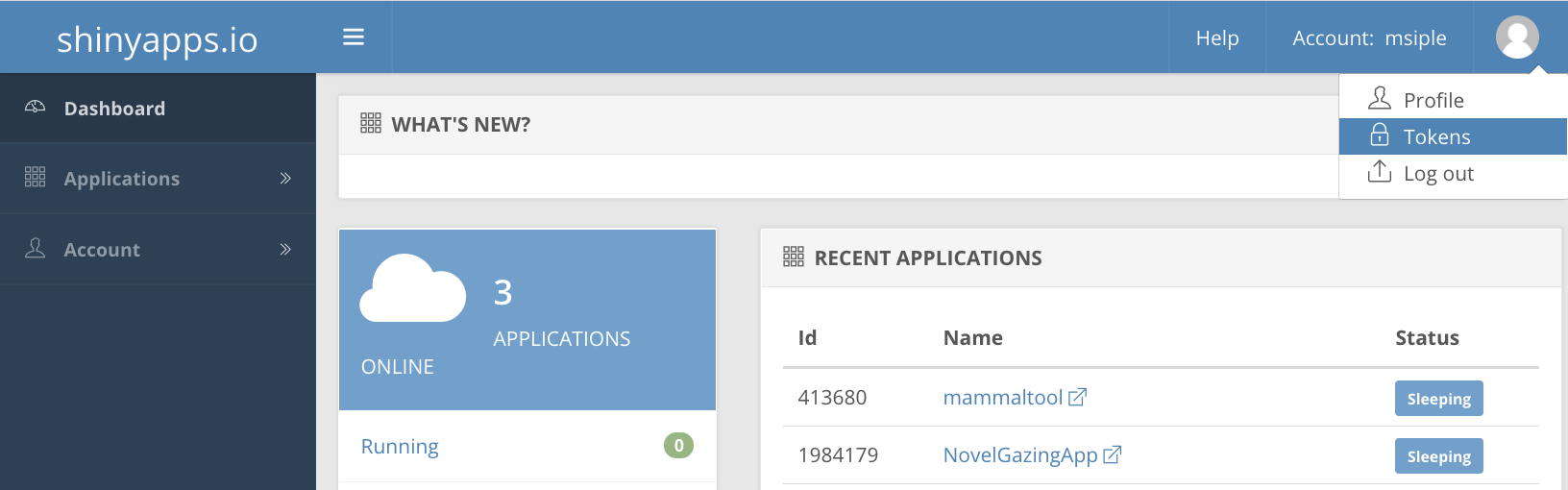 --- # Deploying your Shiny app ## Deploy to the cloud - If you add a new token and click 'show', you'll get instructions for how to configure `rsconnect` - Configure your rsconnect installation. This will link your shinyapps.io account to your local environment: ``` r library(rsconnect) setAccountInfo(name="<ACCOUNT>", token="<TOKEN>", secret="<SECRET>")` ``` - You can deploy from within RStudio by clicking the 'Publish' button in the RStudio IDE, or use the console: ``` r deployApp(server = "shinyapps.io")` ``` - woo! --- # Deploying your Shiny app 2 ## Other options for deployment - Deploy to an RStudio Connect account (local, commercial option) - Publish on Shiny Server (local, open source) --- # Getting credit for your Shiny development If you've put a lot of time into making a Shiny app, you should get credit! The publication/credit avenues are unconventional for Shiny apps, but they DO exist. - Publish as a package in an open source software journal (e.g., [JOSS](https://joss.theoj.org/) ) (check out the `{golem}` pkg for structuring your files and streamlining production) 📦 - Publish code straight from GitHub with a DOI from Zenodo ( [here](https://www.rinproduction.com/en/posts/005-8-tips-for-a-production-ready-shiny-application/) are some good tips for preparing your app for production ) - (for "just for fun" apps): submit your Shiny app to the RStudio [Shiny contest](https://community.rstudio.com/t/shiny-contest-2020-is-here/51002) <!-- see if you can get some data on citation rates for JOSS vs zenodo github entry --> ---